Android Google Map
Android allows us to integrate google maps in our application
You can show any location on the map , or can show different
routes on the map e.t.c. You can also customize the map
according to your choices. Show Place On Your Device
Lets Start Google Map Development App
Google Map Layout
<fragment android:id="@+id/map" android:name="com.google.android.gms.maps.MapFragment" android:layout_width="match_parent" android:layout_height="match_parent"/>
Create Android Application
1. The Application First Step Create a New Project Start project
2. Rename Application Name Below Screen Ex:Google Map
Click Next
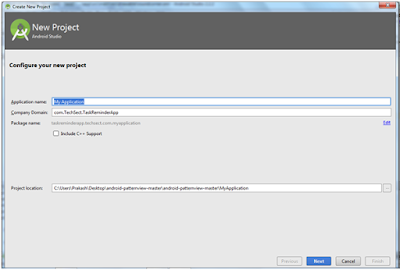
3. After Spacific Minium sdk API 23 Select Lolipop 5.0
Click Next
4. Final Select Google Map Activity And Click Next
5. Then Select MainActivity Click Finish
6. Then Open a Android Studio
1. MapActivity.Java
a. It will open the following screen and copy the console url for API Key as shown below −
Sign In your Gmail Address. Then Paste Your Browser.
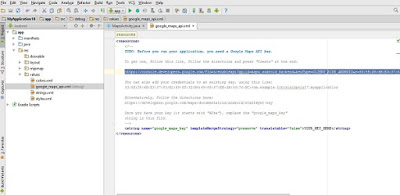
b. Copy this and paste it to your browser. It will give the following screen
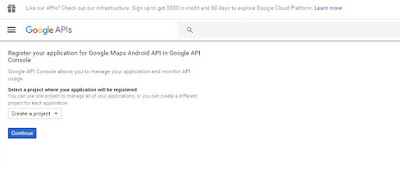
c. Click on continue and click on Create API Key then it will show the following screen
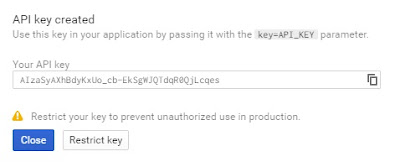
1. MainActivity.java
package com.prakash.googleMap;
import android.support.v4.app.FragmentActivity;
import android.os.Bundle;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.OnMapReadyCallback;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
public class MapsActivity extends FragmentActivity implements OnMapReadyCallback {
private GoogleMap mMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_maps);
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.map);
mapFragment.getMapAsync(this);
}
/**
* Manipulates the map once available.
* This callback is triggered when the map is ready to be used.
* This is where we can add markers or lines, add listeners or move the camera.
* In this case, we just add a marker near Sydney, Australia.
* If Google Play services is not installed on the device.
* This method will only be triggered once the user has installed
Google Play services and returned to the app.
*/
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
// Add a marker in Sydney and move the camera
LatLng TutorialsPoint = new LatLng(21, 57);
mMap.addMarker(new
MarkerOptions().position(TutorialsPoint).title("Tutorialspoint.com"));
mMap.moveCamera(CameraUpdateFactory.newLatLng(TutorialsPoint));
}
}
2. Activity Main.xml
<?xml version="1.0" encoding="utf-8"?>
<?xml version="1.0" encoding="utf-8"?
fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/map"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.tutorialspoint7.myapplication.MapsActivity"/>
3. Activity Mainifests.xml
<?xml version="1.0" encoding="utf-8"?>
<?xml version="1.0" encoding="utf-8"?
<!...;The ACCESS_COARSE/FINE_LOCATION permissions are not required to use
Google Maps Android API v2, but you must specify either coarse or fine
location permissions for the 'MyLocation' functionality.....>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<!...;The API key for Google Maps-based APIs is defined as a string resource
(See the file "res/values/google_maps_api.xml").
Note that the API key is linked to the encryption key used to sign the APK.
You need a different API key for each encryption key, including the release key
that is used to sign the APK for publishing.
You can define the keys for the debug and
release targets in src/debug/ and src/release/......>
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="AIzaSyAXhBdyKxUo_cb-EkSgWJQTdqR0QjLcqes" />
<activity android:name=".MapActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
4.String
<resources>
<string name="app_name">Google Map</string>
<string name="hello_world">Hello world!</string>
<string name="menu_settings">Settings</string>
<string name="title_activity_main">MainActivity</string>
</resources>
5. Output
<< PREVIEW >>
<< NEXT >>
No comments:
Post a Comment